Introduction
On this article, we’re going to discover a brand new software, EmbedAnything, and see the way it works and what you should use it for. EmbedAnything is a high-performance library that means that you can create picture and textual content embeddings straight from recordsdata utilizing native embedding fashions in addition to cloud-based embedding fashions. We may even see an instance of this in motion with a situation the place we wish to group vogue photos collectively primarily based on the attire that they present.
What Are Embeddings?
In case you are working within the AI area or labored with giant language fashions (LLMs), you’d have positively come throughout the time period embeddings. In easy phrases, embeddings are a compressed illustration of a sentence or a phrase. It’s mainly a vector of floating level numbers. It might be of any measurement starting from 100 to as large as 5000.
How Are Embeddings Made?
Embedding fashions have developed loads over time. The earliest fashions had been primarily based on one sizzling encoding or phrase occurrences. Nevertheless, with new technological developments and extra information availability, embedding fashions have grow to be extra highly effective.
Pre-Transformer Period
The best strategy to signify a phrase as an embedding is utilizing a one-hot encoding with the overall vocabulary measurement of the textual content corpus. Nevertheless, that is extraordinarily inefficient because the illustration may be very sparse, and the scale of the embedding is as large because the vocabulary measurement, which might be as much as thousands and thousands.
The following strategy is utilizing an NGram mannequin, which makes use of a easy, totally related neural community. There are two strategies Skip-gram and Steady Bag of Phrases (CBOW). These strategies are very environment friendly and fall beneath the Word2Vec umbrella. CBOW predicts the goal phrase from its context, whereas Skip-gram tries to foretell the context phrases from the goal phrase.
One other strategy is GloVe (International Vectors for Phrase Illustration). GloVe focuses on leveraging the statistical info of phrase co-occurrence throughout a big corpus.
Publish Transformer Period
Bidirectional Encoder Transformer
One of many earliest methods to construct contextual embeddings utilizing transformers was BERT. What’s BERT, should you surprise? It’s a self-supervised approach of predicting masked phrases. It means if we [MASK] one phrase in a sentence, it simply tries to foretell what that phrase might be, and thus, the knowledge strikes each from the left to proper and proper to left of the masked phrase.
Sentence Embeddings
What we have now seen to date are methods to create phrase embeddings. However in lots of instances, we wish to seize a illustration of a sentence as a substitute of simply the phrases within the sentence. There are a number of methods to create sentence embeddings from phrase embeddings. One of the vital prevalent strategies is utilizing pre-trained fashions like SBERT (Sentence BERT). These are educated by making a dataset of comparable pairs of sentences and performing contrastive studying with similarity scores.
There are a number of strategies for utilizing sentence embedding fashions. The simplest is to make use of cloud-based embedding fashions like OpenAI, Jina, or Cohere. There are a number of native fashions as nicely on Hugging Face that can be utilized like AllMiniLM6.
Multimodal Embeddings
Multimodal embeddings are vector representations that encode info from a number of forms of information into a standard vector area. This permits fashions to know and correlate info throughout totally different modalities. One of the vital used multimodal embedding fashions is CLIP, which embeds textual content and pictures in a shared embedding area.
The place Are These Embeddings Used?
Embeddings have a number of purposes throughout varied industries. Listed below are a few of the most typical use instances:
Info Retrieval
- Search Engines: Embeddings are used to enhance search relevance by understanding the semantic which means of queries and paperwork.
- Retrieval Augmented Technology (RAG): Embeddings are used to retrieve data for Massive Language fashions. That is known as LLM Grounding.
- Doc Clustering and Matter Modeling: Embeddings assist in grouping comparable paperwork collectively and discovering latent subjects in a corpus
Multimodal Functions
- Picture Captioning: Combining textual content and picture embeddings to generate descriptive captions for photos.
- Visible Query Answering: Utilizing each visible and textual embeddings to reply questions on photos.
- Multimodal Sentiment Evaluation: Combining textual content, picture, and audio embeddings to research sentiment from multimedia content material.
How Does EmbedAnything Assist?
AI fashions should not straightforward to run. They’re computationally very intensive, not straightforward to deploy, and arduous to observe. EmbedAnything permits you to run embedding fashions effectively and makes them deployment-friendly. Listed below are a few of the advantages of utilizing EmbedAnything enhances the efficiency of AI fashions and might deal with multimodality. When a listing is handed for embedding to EmbedAnything, the file extension is checked to see whether it is textual content or picture and an appropriate embedding mannequin is used to generate the embeddings.
How Does EmbedAnything Work?
EmbedAnything is constructed with Rust. This makes it quicker and supplies sort security and a a lot better improvement expertise. However why is pace so essential on this course of?
Creating embeddings from recordsdata includes two steps that demand vital computational energy:
- Extracting Textual content from Information, Particularly PDFs: Textual content can exist in numerous codecs corresponding to markdown, PDFs, and Phrase paperwork. Nevertheless, extracting textual content from PDFs might be difficult and sometimes causes slowdowns. It’s particularly tough to extract textual content in manageable batches as embedding fashions have a context restrict. Breaking the textual content into paragraphs containing targeted info will help.
- Inferencing on the Transformer Embedding Mannequin: The transformer mannequin is normally on the core of the embedding course of, however it’s identified for being computationally costly. To deal with this, EmbedAnything makes use of the Candle Framework by Hugging Face, a machine-learning framework constructed completely in Rust for optimized efficiency.
The Good thing about Rust for Velocity
By utilizing Rust for its core functionalities, EmbedAnything provides vital pace benefits:
- Rust is Compiled: In contrast to Python, Rust compiles on to machine code, leading to quicker execution.
- Reminiscence Administration: Rust enforces reminiscence administration concurrently, stopping reminiscence leaks and crashes that may plague different languages.
- Rust achieves true multithreading.
What Does Candle Carry to the Desk?
Working language fashions or embedding fashions regionally might be tough, particularly while you wish to deploy a product that makes use of these fashions. In the event you use the transformers library from Hugging Face in Python, you’ll rely upon PyTorch for tensor operations. This, in flip, has a dependency on Libtorch, which suggests that you’ll want to incorporate your complete Libtorch library along with your product. Additionally, Candle permits inferences on CUDA-enabled GPUs proper out of the field. We are going to quickly put up on how we use Candle to extend the efficiency and reduce the reminiscence utilization of EmbedAnything.
The right way to Use EmbedAnything
Let’s have a look at an instance of how handy it’s to make use of EmbedAnything. We are going to have a look at the zero-shot classification of vogue photos. Let’s say we have now some photos like this:
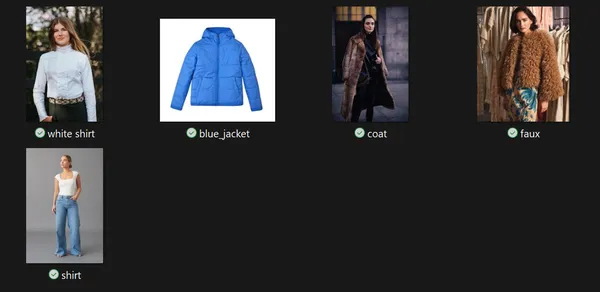
We would like the mannequin to categorize them as [‘Shirt’, ‘Coat’, ‘Jeans’, ‘Skirt’, ‘Hat’, ‘Shoes’, ‘Bag’].
To get began, you’ll want to put in the embed-anything package deal:
pip set up embed-anything
Subsequent, import the mandatory dependencies:
import embed_anything
import numpy as np
from PIL import Picture
import matplotlib.pyplot as plt
With simply two strains of code, you possibly can receive the embeddings for all the photographs in a listing utilizing CLIP embeddings:
information = embed_anything.embed_directory("photos", embeder= "Clip") # embed "photos" folder
embeddings = np.array([data.embedding for data in data])#import csv
Outline the labels that you really want the mannequin to foretell and embed the labels:
labels = ['Shirt', 'Coat', 'Jeans', 'Skirt', 'Hat', 'Shoes', 'Bag']
label_embeddings = embed_anything.embed_query(labels, embeder= "Clip")
label_embeddings = np.array([label.embedding for label in label_embeddings])
fig, ax = plt.subplots(1, 5, figsize=(20, 5))
for i in vary(len(information)):
similarities = np.dot(label_embeddings, information[i].embedding)
max_index = np.argmax(similarities)
image_path = information[i].textual content
# Open and plot the picture
img = Picture.open(image_path)
ax[i].imshow(img)
ax[i].axis('off')
ax[i].set_title(labels[max_index])
That’s it. Now, we simply verify the similarities between the picture embeddings and the label embeddings and assign the label to the picture with the best similarity. We will additionally visualize the output.
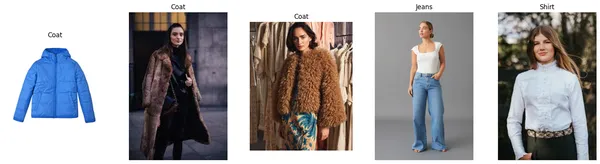
Conclusion
With EmbedAnything, including extra photos to the folder or extra labels to the record is easy. This technique scales very nicely and doesn’t require any coaching, making it a strong software for zero-shot classification duties
On this article, we discovered about embedding fashions and the right way to use EmbedAnything to boost your embedding pipeline, dashing up the era course of with only a few strains of code.
You possibly can take a look at EmbedAnything, right here.
We’re actively on the lookout for contributors to construct and prolong the pipeline to make embeddings simpler and extra highly effective.