Introduction
In arithmetic, we use exponents or powers to display the repeated multiplication of a quantity by itself. Python provides a number of methods to perform exponentiation, offering simplicity and flexibility for a spread of use conditions. Python gives highly effective instruments to carry out a variety of duties, from simple arithmetic operations to intricate scientific calculations. You may entry these capabilities by the ** operator, the maths.pow() perform from the math module, or the built-in pow() perform. On this article we are going to look into the element about exponents in Python. We may also find out about superior exponentiation strategies, optimizations and greatest practices and the frequent pitfall additionally.
Fundamental Strategies to Calculate Exponents in Python
In arithmetic, we basically use exponents, also called powers, and we are able to compute them utilizing varied strategies in Python. The **
operator, the pow() perform, and math.pow() from the maths module are generally used to carry out exponentiation, offering flexibility and precision in several situations.
Utilizing the **
Operator
The **
operator is essentially the most easy technique to carry out exponentiation in Python. It really works for each integers and floating-point numbers.
base = 3
exponent = 4
end result = base ** exponent
print(f"{base} to the facility of {exponent} is {end result}")
# Output: 3 to the facility of 4 is 81
Utilizing the pow() Perform
You should use the built-in pow() perform for exponentiation, and it gives a further third parameter for modular arithmetic.
base = 5
exponent = 3
end result = pow(base, exponent)
print(f"{base} to the facility of {exponent} is {end result}")
# Output: 5 to the facility of three is 125
Utilizing the maths.pow() Perform
The maths.pow() perform from the maths module is particularly for floating-point exponentiation and returns a float.
import math
base = 2
exponent = 8
end result = math.pow(base, exponent)
print(f"{base} to the facility of {exponent} is {end result}")
# Output: 2 to the facility of 8 is 256.0
Superior Exponentiation Strategies
Python superior exponentiation strategies embody dealing with fractional and unfavourable exponents, successfully dealing with big computations, and investigating exponentiation of complicated numbers. These strategies make use of Python’s adaptability and built-in capabilities to handle quite a lot of mathematical duties.
Detrimental and Fractional Exponents
Python helps unfavourable and fractional exponents, permitting for calculations of reciprocals and roots.
base = 16
negative_exponent = -2
fractional_exponent = 0.5
result_negative = base ** negative_exponent
result_fractional = base ** fractional_exponent
print(f"{base} to the facility of {negative_exponent} is {result_negative}")
print(f"{base} to the facility of {fractional_exponent} is {result_fractional}")
# Output: 16 to the facility of -2 is 0.00390625
# 16 to the facility of 0.5 is 4.0
Dealing with Massive Exponents
Massive exponent calculations may end up in very giant numbers. Python’s integer sort can deal with arbitrarily giant values, however it is best to take into account efficiency and reminiscence utilization.
large_base = 2
large_exponent = 1000
end result = large_base ** large_exponent
print(f"{large_base} to the facility of {large_exponent} has {len(str(end result))} digits")
#output: 2 to the facility of 1000 has 302 digits
Complicated Quantity Exponentiation
Utilizing the cmath module, Python can deal with complicated quantity exponentiation.
import cmath
base = complicated(1, 2)
exponent = 3
end result = base ** exponent
print(f"({base}) to the facility of {exponent} is {end result}")
# Output: ((1+2j)) to the facility of three is (-11+2j)
Sensible Functions of Exponents
Folks use Python, a versatile program, in pc science, physics, economics, finance, and different fields for scientific and computational functions involving exponents. With the usage of its sturdy instruments, one might compute compound curiosity, simulate development and decay processes, and resolve difficult mathematical points.
Progress Calculations (e.g., compound curiosity)
When computing compound curiosity, the place the quantity will increase exponentially over time, exponents play a essential function.
principal = 1000
price = 0.05
times_compounded = 4
years = 5
quantity = principal * (1 + price/times_compounded) ** (times_compounded * years)
print(f"Quantity after {years} years: {quantity}")
# Output: Quantity after 5 years: 1283.36
Scientific Calculations (e.g., exponential decay)
Exponential decay describes processes that lower quickly at first after which stage off. It’s generally utilized in science and engineering.
initial_amount = 100
decay_constant = 0.1
time = 10
remaining_amount = initial_amount * math.exp(-decay_constant * time)
print(f"Remaining quantity after {time} models of time: {remaining_amount}")
# Output: Remaining quantity after 10 models of time: 36.79
Pc Graphics and Animations
In pc graphics, exponential capabilities are used to simulate quite a lot of pure occurrences and animations.
import numpy as np
import matplotlib.pyplot as plt
x = np.linspace(0, 10, 100)
y = np.exp(x)
plt.plot(x, y)
plt.title('Exponential Progress')
plt.xlabel('x')
plt.ylabel('exp(x)')
plt.present()
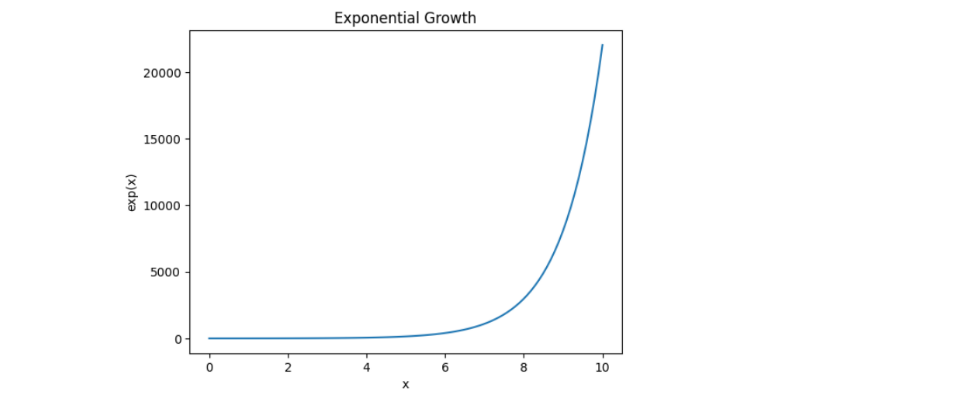
Optimizations and Greatest Practices
Contemplate optimizing greatest practices and using these pointers and techniques to leverage the benefits of exponentiation with a purpose to produce correct and environment friendly calculations with exponents in Python.
Selecting the Proper Technique
Utilizing the **
Operator for Simplicity:
- The
**
operator is concise and straightforward to make use of for general-purpose exponentiation. - It’s appropriate for most elementary calculations involving integers and floats.
base = 3
exponent = 4
end result = base ** exponent
print(end result) # Output: 81
Use pow()
for Compatibility and Modulo Operations:
- The built-in pow() perform is helpful if you want compatibility with different built-in capabilities or when performing modular exponentiation.
- Modular exponentiation (i.e., (a ** b) % c) might be carried out effectively utilizing the third argument of pow().
base = 5
exponent = 3
mod = 13
end result = pow(base, exponent, mod)
print(end result) # Output: 8
Use math.pow() for Floating-Level Exponentiation:
- It’s particularly designed for floating-point calculations and all the time returns a float.
- This perform is a part of the maths module, which incorporates many different mathematical capabilities that may be helpful in scientific and engineering functions.
import math
base = 2.0
exponent = 3.0
end result = math.pow(base, exponent)
print(end result) # Output: 8.0
Efficiency Concerns
Use numpy
for Massive-Scale Numerical Computations:
- The numpy library gives optimized capabilities for numerical operations, together with exponentiation.
- It’s extremely environment friendly for operations on giant arrays and matrices, making it supreme for scientific computing and knowledge evaluation.
import numpy as np
large_base = np.array([2])
large_exponent = 1000
end result = np.energy(large_base, large_exponent)
print(end result) # Output: [10715086071862673209484250490600018105614048117055336074437503883703510511249361224931983788156958581275946729175531468251871452856923140435984577574698574803934567774824230985421074605062371141877954182153046474983581941267398767559165543946077062914571196477686542167660429831652624386837205668069376]
Precompute Exponents When Attainable:
- If it’s good to carry out the identical exponentiation a number of instances, take into account precomputing the end result and storing it in a variable.
- This could save computation time and enhance efficiency, particularly in loops or recursive capabilities.
base = 2
exponent = 10
precomputed_result = base ** exponent
for _ in vary(1000):
end result = precomputed_result
# Carry out different operations utilizing the end result
Reminiscence Utilization and Effectivity
Keep away from Pointless Floating-Level Conversions:
- Use integer exponentiation when potential to keep away from the overhead and potential precision points related to floating-point arithmetic.
- Reserve math.pow() and related capabilities for instances the place floating-point precision is required.
# Integer exponentiation
base = 3
exponent = 10
end result = base ** exponent # Extra environment friendly than utilizing math.pow()
Deal with Massive Numbers Rigorously:
- Python’s int sort can deal with arbitrarily giant numbers, however operations on very giant numbers might be sluggish and memory-intensive.
- For terribly giant numbers, think about using specialised libraries equivalent to gmpy2 for sooner efficiency.
import gmpy2
base = gmpy2.mpz(2)
exponent = 1000
end result = gmpy2.powmod(base, exponent, gmpy2.mpz(10**10))
print(end result)
Greatest Practices for Writing Environment friendly Code
Writing environment friendly code for exponentiation in Python entails selecting the best technique (**
operator, pow(), math.pow()), optimizing for efficiency utilizing libraries like numpy, dealing with edge instances rigorously (floating-point precision, overflow errors), and utilizing capabilities and modules for higher group and reusability.
Use Features and Modules
- Encapsulate repeated exponentiation logic in capabilities to enhance code readability and maintainability.
- Use Python’s commonplace libraries and third-party modules for well-tested and optimized implementations.
def calculate_exponent(base, exponent):
return base ** exponent
end result = calculate_exponent(3, 4)
print(end result) # Output: 81
Profile and Optimize
- Make use of profiling utilities like as timeit and cProfile to find efficiency bottlenecks in your code.
- Solely these sections of your code that considerably have an effect on efficiency ought to be optimized.
import timeit
setup = "base = 2; exponent = 1000"
assertion = "end result = base ** exponent"
time_taken = timeit.timeit(stmt=assertion, setup=setup, quantity=100000)
print(f"Time taken: {time_taken} seconds")
Widespread Pitfalls and Keep away from Them
Understanding frequent pitfalls in Python when working with exponents is essential for writing sturdy and environment friendly code, as they will result in incorrect outcomes or efficiency points.
Coping with Floating-Level Precision
Floating-point numbers are represented in a approach that may result in precision points, particularly when coping with very giant or very small numbers. This could trigger inaccuracies in calculations. As an illustration:
end result = 10 ** 20
print(end result) # Output: 100000000000000000000
end result = math.pow(10, 20)
print(end result) # Output: 1e+20
To keep away from these points, you should utilize the decimal module for arbitrary precision arithmetic when excessive precision is required:
from decimal import Decimal, getcontext
getcontext().prec = 50 # Set precision to 50 decimal locations
base = Decimal(2)
exponent = Decimal(1000)
end result = base ** exponent
print(end result)
Avoiding Overflow Errors
Exponentiation may end up in extraordinarily giant numbers, resulting in overflow errors in fixed-precision methods or efficiency and reminiscence points even in arbitrary-precision methods like Python’s int. Right here’s an instance:
attempt:
end result = 2 ** 10000
besides OverflowError as e:
print(f"OverflowError: {e}")
To deal with giant numbers successfully, use acceptable knowledge sorts and algorithms, and think about using modular arithmetic to maintain numbers manageable:
import gmpy2
base = gmpy2.mpz(2)
exponent = 1000
modulus = gmpy2.mpz(10**10)
end result = gmpy2.powmod(base, exponent, modulus)
print(end result)
Dealing with Detrimental and Fractional Exponents
Detrimental and fractional exponents can result in surprising outcomes or errors, particularly when used with integer bases. For instance:
base = 16
negative_exponent = -2
fractional_exponent = 0.5
result_negative = base ** negative_exponent
result_fractional = base ** fractional_exponent
print(result_negative) # Output: 0.00390625
print(result_fractional) # Output: 4.0
Be sure that the bottom and exponent sorts are acceptable for the operation and use the maths module capabilities when coping with non-integer exponents:
import math
base = 16
fractional_exponent = 0.5
end result = math.pow(base, fractional_exponent)
print(end result) # Output: 4.0
Efficiency Points with Massive-Scale Exponentiation
Exponentiation with giant bases and exponents might be computationally costly and memory-intensive, resulting in efficiency bottlenecks. As an illustration:
base = 2
exponent = 100000
end result = base ** exponent # This may be sluggish and memory-intensive
Use optimized libraries like numpy
for large-scale numerical computations:
import numpy as np
large_base = np.array([2])
large_exponent = 1000
end result = np.energy(large_base, large_exponent)
print(end result)
Incorrect Use of Features
Utilizing the incorrect perform for a particular sort of exponentiation can result in incorrect outcomes or decreased efficiency. For instance:
end result = 2 ** 3 ** 2 # That is interpreted as 2 ** (3 ** 2)
print(end result) # Output: 512
Use parentheses to explicitly outline the order of operations:
end result = (2 ** 3) ** 2
print(end result) # Output: 64
Conclusion
Python’s builders have strategically constructed sturdy exponentiation capabilities into the language, offering customers with a flexible toolkit for mathematical computations, that includes a spread of approaches, from easy ** operator utilization to superior unfavourable exponents and sophisticated quantity operations. Builders might absolutely make the most of exponentiation in Python to allow exact and environment friendly options in quite a lot of scientific, monetary, and computational domains by following optimization methodologies, using related modules, and avoiding frequent errors.
Don’t miss this opportunity to develop your talents and additional your profession. Come be taught Python with us! We provide an intensive and charming free course that’s appropriate for all ranges.